반응형
Swiper ✨
1. Swiper 란?
Swiper는 하드웨어 가속 전환과 놀라운 네이티드 동작을 갖춘 무료 오픈 소스 슬라이더입니다.
사용 이유 ✨
1. 간단한 사용법
HTML, CSS, JS 코드로 반응형 슬라이드쇼 및 스와이퍼 기능을 쉽게 구현할 수 있어서 사용하게 되었습니다!
2. 다양한 슬라이드 유형
Swiper는 일반적인 이미지 슬라이드뿐만 아니라 텍스트, 비디오, 오디오 등 다양한 유형의 슬라이드를 지원합니다!
3. 반응형 디자인
반응형을 지원하기 때문에 데스크탑, 태블릿, 모바일 기기에서 사용자 경험을 제공할 수 있습니다!
사용 방법 📖
1. NPM에서 설치
- NPM에서 Swiper를 설치할 수 있습니다.
$ npm install swiper
- 기본적으로 Swiper는 추가 모듈 없이 핵심 버전만 내보내기 때문에 Navigation, Pagination 등을 따로 구성해야 됩니다.
// import Swiper JS
import Swiper from 'swiper';
// import Swiper styles
import 'swiper/css';
const swiper = new Swiper(...);
2. CDN을 이용한 방법
- CDN을 이용한 방법
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/swiper@11/swiper-bundle.min.css"/>
<script src="https://cdn.jsdelivr.net/npm/swiper@11/swiper-bundle.min.js"></script>
3. 로컬을 이용한 방법
- Swiper 에셋을 다운받아 사용할 수 있습니다.
<link rel="stylesheet" href="path/to/swiper-bundle.min.css" />
<script src="path/to/swiper-bundle.min.js"></script>
4. 기본 설정
- head 태그에 Swiper 라이브러리와 스타일을 로드합니다.
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/swiper@11/swiper-bundle.min.css"/>
<script src="https://cdn.jsdelivr.net/npm/swiper@11/swiper-bundle.min.js"></script>
- html 파일에 기본 Swiper 레이아웃을 설정합니다.
<!-- Slider main container -->
<div class="swiper">
<!-- Additional required wrapper -->
<div class="swiper-wrapper">
<!-- Slides -->
<div class="swiper-slide">Slide 1</div>
<div class="swiper-slide">Slide 2</div>
<div class="swiper-slide">Slide 3</div>
...
</div>
<!-- If we need pagination -->
<div class="swiper-pagination"></div>
<!-- If we need navigation buttons -->
<div class="swiper-button-prev"></div>
<div class="swiper-button-next"></div>
</div>
- 스와이퍼 초기화
const swiper = new Swiper('.swiper', {
// Optional parameters
direction: 'vertical',
loop: true,
// If we need pagination
pagination: {
el: '.swiper-pagination',
},
// Navigation arrows
navigation: {
nextEl: '.swiper-button-next',
prevEl: '.swiper-button-prev',
},
});
5. 내가 작성한 코드
- HTML
<div class="swiper">
<div class="swiper-wrapper">
<div class="swiper-slide">
<img src="./images/이석원.png" alt="이석원 각오">
</div>
<div class="swiper-slide">
<img src="./images/유인철.png" alt="유인철 각오">
</div>
<div class="swiper-slide">
<img src="./images/김경혜.png" alt="김경혜 각오">
</div>
<div class="swiper-slide">
<img src="./images/이원빈.png" alt="이원빈 각오">
</div>
<div class="swiper-slide">
<img src="./images/이예람.png" alt="이예람 각오">
</div>
</div>
<div class="swiper-pagination"></div>
<div class="swiper-button-prev"></div>
<div class="swiper-button-next"></div>
</div>
- CSS
/* 이미지 슬라이드 */
.swiper {
max-width: 800px;
height: 324px;
overflow: visible;
}
.swiper-slide {
display: flex;
justify-content: center;
align-items: center;
overflow: visible;
}
.swiper-slide img {
width: 659px;
height: 281px;
max-width: 659px;
object-fit: cover;
}
.swiper {
--swiper-theme-color: grey;
--swiper-pagination-bullet-size: 10px;
--swiper-pagination-bullet-inactive-color: #D9D9D9;
--swiper-pagination-bullet-inactive-opacity: 0.4;
--swiper-navigation-color: #FF6C7A;
}
- JS
// 이미지 슬라이드
const swiper = new Swiper(".swiper", {
direction: "horizontal",
loop: "infinite",
autoplay: {
delay: 2000,
},
// Pagination
pagination: {
el: ".swiper-pagination",
clickable: true,
},
// Navigation arrows
navigation: {
nextEl: ".swiper-button-next",
prevEl: ".swiper-button-prev",
},
});
옵션 📖
1. 사용한 옵션
기본 옵션은 공식 홈페이지에 정~~말 많습니다! 참고 하세용!
이름 | 유형 | 기본 | 설명 |
direction | horizontal, vertical | horizontal | 수평 또는 수직 설정 horizontal: 수평 방향 vertical: 수직 방향
|
loop | boolean | false | 연속 루프 모드를 활성화 하려면 값을 true로 설정해야 합니다. true: 무한 루프(infinite) false: 루프 없음
|
autoplay | any | 자동 재생을 설정하려면 값을 true로 설정해야 합니다. false: 자동 재생 끔 true: 자동 재생 켬 delay: 자동 재생 딜레이 시간(ms)
|
|
pagination | any |
|
|
navigation | any |
|
깨달은 점 ❕
1. 사용자 정의 CSS
사용자 정의 CSS를 지원해주기 때문에 navigation, pagination의 CSS 속성을 정말 간편하게 수정할 수 있습니다!
{
/* navigation */
--swiper-navigation-size: 44px;
--swiper-navigation-top-offset: 50%;
--swiper-navigation-sides-offset: 10px;
--swiper-navigation-color: var(--swiper-theme-color);
/* pagination */
--swiper-pagination-color: var(--swiper-theme-color);
--swiper-pagination-left: auto;
--swiper-pagination-right: 8px;
--swiper-pagination-bottom: 8px;
--swiper-pagination-top: auto;
--swiper-pagination-fraction-color: inherit;
--swiper-pagination-progressbar-bg-color: rgba(0, 0, 0, 0.25);
--swiper-pagination-progressbar-size: 4px;
--swiper-pagination-bullet-size: 8px;
--swiper-pagination-bullet-width: 8px;
--swiper-pagination-bullet-height: 8px;
--swiper-pagination-bullet-inactive-color: #000;
--swiper-pagination-bullet-inactive-opacity: 0.2;
--swiper-pagination-bullet-opacity: 1;
--swiper-pagination-bullet-horizontal-gap: 4px;
--swiper-pagination-bullet-vertical-gap: 6px;
}
2. 공식 문서
공식 문서가 정말 잘 나와 있습니다!
그래서 업데이트 로그들을 확인해서 버그 수정, 성능 개선 사항들을 확인할 수 있는 점도 좋은 것 같습니다!
11.1.5 (2024-07-15)
Bug Fixes
- element: fix observer to watch for slides (7cffede), closes #7598
- improved 3d rotate fix in Safari (cb83879), closes #7532
- update navigation.scss to remove SASS Deprecation Warning (#7612) (a3e0bf8)
- vue: add breakpointsBase type (4adb85b), closes #7607
가장 최신 버전 업데이트가 7/15입니다! 버그 수정이 된 따끈따끈한 로그가 올라왔으니 확인해 보시는 것도 추천드립니다!
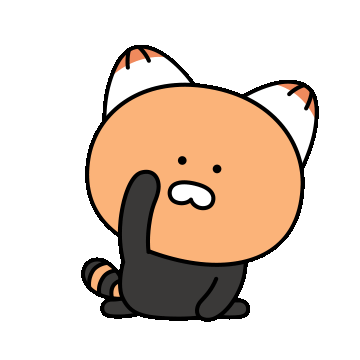
출처 🏷️
Swiper - The Most Modern Mobile Touch Slider
Swiper is the most modern free mobile touch slider with hardware accelerated transitions and amazing native behavior.
swiperjs.com
반응형
'공부 > TIL' 카테고리의 다른 글
옵저버 패턴 알아보기 (0) | 2024.09.10 |
---|---|
[TIL] 24.07.17 쓰로틀링(성능 측정) (0) | 2024.07.17 |
[TIL] 24.07.16 코딩 컨벤션이란? (0) | 2024.07.16 |
[TIL] 24.07.15 (0) | 2024.07.15 |
[TIL] 24.07.09 백준 10844번 js (0) | 2024.07.09 |
[TIL] 24.07.08 (0) | 2024.07.08 |
[TIL] 24.06.17 백준 1373번 js (0) | 2024.06.17 |
[TIL] 24.06.12 백준 1676번 js (2) | 2024.06.12 |